Arduino 433 MHz Wireless Module
·
Timo Denk
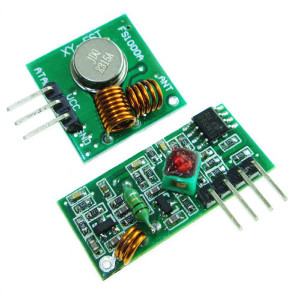
This post provides a quick reference for the 433 MHz wireless module, which is widely used in combination with the Arduino. The VirtualWire library is one possible way of utilizing the module.
Before you get started make sure you have attached antennas to both, transmitter and receiver. The wavelength can be calculated using the following formula.
$$\lambda = \frac{c}{f}$$
For $f=433\text{ MHz}$ this leads to $\lambda = 0.6923\text{ m}$. A monopole antenna would usually have a length of $l=\frac{1}{4}\lambda$ or $l=\frac{3}{2}\lambda$.
Wiring
Transmitter
VCC | 3.5 – 12 V | Power supply |
---|---|---|
ATAD | Arduino pin 12 | Serial data |
GND | GND | Ground (0 V) |
Receiver
VCC | 5 V | Power supply |
---|---|---|
DATA | Arduino pin 12 | Serial data |
GND | GND | Ground (0 V) |
Code
Transmitter
// library #include <VirtualWire.h> void setup() { Serial.begin(9600); // virtual wire vw_set_tx_pin(12); // pin vw_setup(8000); // bps } void loop() { sendString("message", true); delay(100); } void sendString(String message, bool wait) { byte messageLength = message.length() + 1; // convert string to char array char charBuffer[messageLength]; message.toCharArray(charBuffer, messageLength); vw_send((uint8_t *)charBuffer, messageLength); if (wait) vw_wait_tx(); Serial.println("sent: " + message); }
Receiver
// library #include <VirtualWire.h> byte message[VW_MAX_MESSAGE_LEN]; // a buffer to store the incoming messages byte messageLength = VW_MAX_MESSAGE_LEN; // the size of the message void setup() { Serial.begin(9600); Serial.println("device is ready..."); vw_set_rx_pin(12); // pin vw_setup(8000); // bps vw_rx_start(); } void loop() { if (vw_get_message(message, &messageLength)) // non-blocking { Serial.print("received: "); for (int i = 0; i < messageLength; i++) { Serial.write(message[i]); } Serial.println(); } }
Range Extension
There are three primary factors affecting the overall range. With optimisation one can get ranges of up to 150 m (source).
- Antenna. Make sure you are using an adequate antenna, ideally one that is distinguished to be working with the frequency of your module.
- Transmitter voltage. The higher the transmitter voltage, the stronger the signal. For maximum range the voltage can be up to $U=12\text{ V}$.
- Transmission speed. Lower transmission speeds increase the transmission range. You can decrease the bits-per-second (bps) rate by changing the lines
vw_setup(8000);
in the transmitter and the receiver code. For a long range a value of approximately 500 bps is suitable.