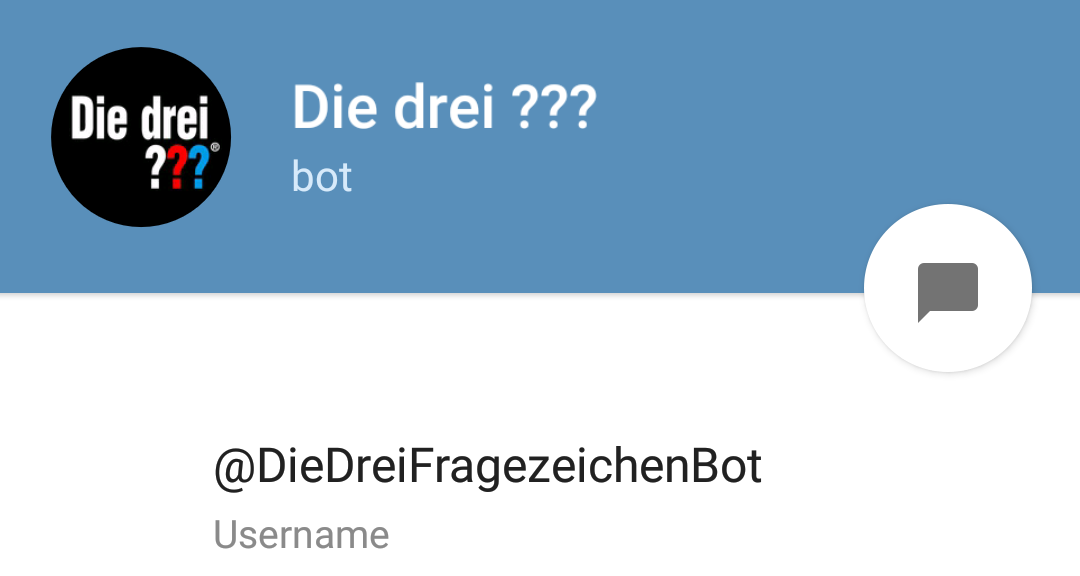
Die drei ??? Telegram Bot
Since the publisher Europa uploads new Die drei ??? episodes on Spotify quite frequently, I have been wanting to get an update every time they upload something new. For me the most convenient way to be notified is to get a message on my smartphone. The free messenger Telegram is predestinated for that task because of its open API and bot capabilities.
A bot is like a Telegram user. The only difference: You are not chatting with a person but a program that is running on a server somewhere else. For my bot that is a Node.js script, being executed on my Raspberry Pi.
How does it Work?
To get updates you have to search for the bot on Telegram (the name is @DieDreiFragezeichenBot) or click on that link: @DieDreiFragezeichenBot
Then you have to subscribe by writing /start
. The bot will respond with a success message. Now you are on a list of subscribed clients and the next time the bot detects new episodes, you will get an update pretty much like that:
Commands
There are some other commands available:
/start
to subscribe to updates/stop
to unsubscribe from any updates/help
to get basic information about the bot/debug
to get your chat ID (for debugging purposes)/random
to get the link to a random episode
The Technology Behind
The whole system is a Node.js application. Exciting about it is that it was written in TypeScript (my first TypeScript project by the way), meaning it uses classes and other common OOP-features, that JavaScript does not offer.
The Telegram API does not allow bots to send too many messages per second (approximately 15 – more details on the Telegram website). Since I was hitting that limit quickly I had to adjust my Telegram.Bot
class to have an outbox (private outbox: Message[] = new Array<Message>();
) containing messages. When another part of the app calls the sendMessage(chatID: number, msg: string)
function, the bot does not send the message immediately. There is another layer below ensuring that the number of outgoing messages per second is limited. If many messages are being sent at the same time, some of them will be stalled and transferred with a short delay.
TypeScript’s object oriented features are generally quite helpful. It is easy to write clean reusable code. This is for example the Spotify.Artist
class that contains a small bit of functionality:
export class Artist { private albums: Album[] = new Array<Album>(); constructor(private artistID: string) { } public getAlbums(): Album[] { return this.albums; } public getRandomAlbum(): Album { let index: number = Math.floor(Math.random() * this.albums.length); return this.albums[index]; } public getID(): string { return this.artistID; } public async downloadAlbums() { let downloader: AlbumArrayDownloader = new AlbumArrayDownloader(this.artistID); try { this.albums = await downloader.run(); } catch (error) { console.log(error); } } }
You can find the entire source code on GitHub Simsso / Die-drei-Fragezeichen-Telegram-bot.
Platform
The used Node module, responsible for the communication with Telegram’s API is called telegram-node-bot. It makes use of ECMAScript 6 which in turn requires at least Node.js version 6. The problem that I ran into was that it is not possible to install Node v6 on the first two Raspberry Pi Revisions. On the newest Raspberry Pi (Rev 3) it works perfectly fine since it has an ARMv8{.mw-redirect} chip architecture.
The app can also be executed on a Mac or Windows machine but for the application a 24/7 up-time is pretty much a requirement. That is why I got the new Raspberry Pi 3 to develop and execute right there.